セマンティック信頼度
注釈
この機能は、実験的な機能であり、変更される可能性があります。また、サポート対象外のため、本番環境には使用しないでください。これらの実験的な機能は、今後のリリースで提供される可能性のあるプレビュー版として提供されています。実験的機能に関するフィードバックは、Lightshipの開発者コミュニティ に投稿してください。
セマンティック信頼度は、ARDKの セマンティックセグメンテーション の機能を拡張するアップデートです。セマンティック信頼度を使用して、所定のカメラフレームのピクセルがセマンティッククラスに属する可能性を判断します。たとえば、あるピクセルが「空」を表す可能性が80%、「葉」を表す可能性が30%であるとします。
セマンティック信頼度は、ARDKのセマンティック処理に可視性の追加レイヤーを提供します。特定のチャンネルに対する特定のレベルの信頼度が必要な場合、またはピクセルが表す可能性のあるチャンネルについてより詳細な情報が必要な場合に、セマンティック信頼度を使用します。たとえば、セマンティック境界間のエッジを滑らかにブレンドし、マスキングにセマンティックを使用する際にビジュアルエイリアシングを減らすために、セマンティック信頼度値を使用することができます。あるいは、特定のチャンネルに含まれる可能性が75%以上のピクセルのみを使用することもできます。
以下のクリップは、既存のARDKのしきい値設定されたセマンティックセグメンテーションデータ(真ん中のクリップ)とセマンティック信頼度データ(右端のクリップ)の違いを示しています。
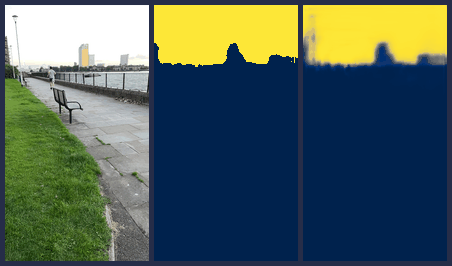
セマンティック信頼度の生成には、セマンティックセグメンテーションと同じ デバイスとOSの要件 が設定されています。
信頼度データにアクセスする
セマンティック信頼度データは、 ARSemanticSegmentationManager を使用するか、 IARFrame からアクセスすることができます。セマンティック信頼度データは、ARDKですでに取得した、しきい値設定済みのセマンティックセグメンテーションデータとは別に提供されます。セマンティック信頼度データには、 ISemanticBuffer インターフェイスからアクセスすることはできません。しきい値設定済みのセマンティックデータのみが、 ISemanticBuffer
によって提供されます。
セマンティックチャンネルの信頼度は、0.0(0%の可能性)から1.0(100%の可能性)までのピクセル単位の浮動小数で表されます。
ARSemanticSegmentationManagerを使用してアクセスする
ARSemanticSegmentationManager
を使用すると、セマンティック信頼度データを収集するセマンティックチャンネルを設定し、そのチャンネルに対する信頼度値を問い合わせることができます。
ARDKで信頼度値を取得するチャンネルを指定するには、チャンネル名の配列を ARSemanticSegmentationManager.SetConfidenceChannels() に渡します。
using Niantic.ARDK.AR;
using Niantic.ARDK.AR.Awareness;
using Niantic.ARDK.AR.Awareness.Semantics;
using Niantic.ARDK.Extensions;
private ARSemanticSegmentationManager _semanticSegmentationManager;
// Channels we want to get semantic confidence data for
private readonly string[] _channels = {
"artificial_ground", "sky", "building", "person"
};
private void Start()
{
// Tell the manager what channels does it need to maintain confidences for
_semanticSegmentationManager.SetConfidenceChannels(_channels);
// Set event handler for semantic buffer update
_semanticSegmentationManager.SemanticBufferUpdated += OnSemanticBufferUpdated;
}
セマンティック信頼度データを取得するには、 ARSemanticSegmentationManager.SemanticBufferUpdated のイベントハンドラを追加し、イベントハンドラで GetConfidences() を使用して特定のチャンネルの信頼度値バッファ値を取得するか、 GetConfidencesRFloat() または GetConfidencesARGB32() を使用して、特定のチャンネル向けに float
または ARGB Texture2D
を作成します。
次の例では、特定のチャンネルのセマンティック信頼度のARGB Texture2D
を取得し、ディスプレイにレンダリングしています。
using Niantic.ARDK.AR;
using Niantic.ARDK.AR.Awareness;
using Niantic.ARDK.AR.Awareness.Semantics;
using Niantic.ARDK.Configuration;
using Niantic.ARDK.Extensions;
using Niantic.ARDK.Rendering;
private ARSemanticSegmentationManager _semanticSegmentationManager;
private Texture2D _semanticTexture;
private Material _overlayMaterial;
// The index of the currently displayed semantics channel in the array
private int _featureChannel;
// Channels we care about in this example
private readonly string[] _channels = {
"artificial_ground", "sky", "building", "person"
};
// Handle SemanticBufferUpdated event to get confidence values into texture
private void OnSemanticBufferUpdated(ContextAwarenessStreamUpdatedArgs<ISemanticBuffer> args)
{
if (!args.IsKeyFrame)
{
return;
}
// Get the name of the observed channel
var currentChannelName = _channels[_featureChannel];
// Update the texture using confidence values
_semanticSegmentationManager.GetConfidencesARGB32
(
ref _semanticTexture,
currentChannelName
);
}
// We use this callback to overlay semantics on the rendered background
private void OnRenderImage(RenderTexture src, RenderTexture dest)
{
// Get the transformation to correctly map the texture to the viewport
var sampler = _semanticSegmentationManager.SemanticBufferProcessor.SamplerTransform;
// Update the transform
_overlayMaterial.SetMatrix(PropertyBindings.SemanticsTransform, sampler);
// Update the texture
_overlayMaterial.SetTexture(PropertyBindings.SemanticChannel, _semanticTexture);
// Display semantics
Graphics.Blit(src, dest, _overlayMaterial);
}
IARFrameを使用してアクセスする
現在のフレームのセマンティック信頼度データは、 IARFrame.CopySemanticConfidences() メソッドを使用して取得できます。このメソッドでは、特定のセマンティックチャンネルに対するピクセル単位の信頼性値の浮動小数点バッファが返ります。また、 AwarenessUtils 拡張を使用して信頼度データを取得することもできます。信頼度データの浮動小数またはARGB Texture2D表現を取得するには、 AwarenessUtils
の CopySemanticConfidencesRFloat() メソッド、または CopySemanticConfidencesARGB32() メソッドを使用します。
using Niantic.ARDK.AR;
using Niantic.ARDK.Extensions;
using Niantic.ARDK.AR.ARSessionEventArgs;
using Niantic.ARDK.AR.Awareness;
using Niantic.ARDK.AR.Awareness.Semantics;
private Texture2D _semanticTexture;
private Matrix4x4 _samplerTransform;
private void ARSession_OnFrameUpdated(FrameUpdatedArgs args)
{
var frame = args.Frame;
if (frame == null || frame.Semantics == null)
return;
frame.CopySemanticConfidencesARGB32
(
"sky",
desired_width,
desired_height,
ref _semanticTexture,
out var sampler
);
// Use sampler transform in your OnRenderImage() as needed
_samplerTransform = sampler;
}
最新のフレームを取得するには、 IARSession.CurrentFrame プロパティを使用するか、 IARSession.FrameUpdated イベントをサブスクライブします。
その他の使用例
その他のコード例は、 ContextAwareness/Segmentation
の ARDK使用例 のUnityのSemanticSegmentationサンプルシーンの SemanticSegmentationExampleManager
を参照してください。