How to Exclude Semantic Channels with Mesh Filtering
Semantic Mesh Filtering allows you to set an allow list or block list of semantic channels. These follow standard allow/block list behavior. Using an allow list will exclude all channels not in the list, while the block list excludes all channels in the list.
For a list of usable semantic channels, see Semantics.
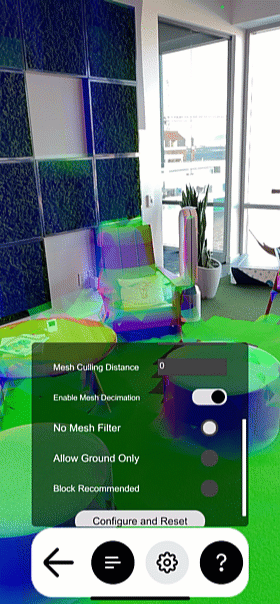
Prerequisites
You will need a Unity project with ARDK installed and a basic AR scene. For more information, see Installing ARDK 3 and Setting up an AR Scene.
Your project must also have the Lightship Meshing subsystem. To add Meshing to your project, follow the steps under Creating the Mesh.
Setting Up Semantic Mesh Filtering
To set up semantic mesh filtering:
- In the Hierarchy, expand the XROrigin and Camera Offset, then select the Main Camera.
- In the Inspector, click Add Component, then add an
ARSegmentationManager
to the Main Camera. - In the Hierarchy, select the MeshManager
GameObject
that you created during the Meshing setup. - Next, find the Lightship Meshing Extension, then check the box next to Mesh Filtering to enable it.
- Two options will appear: Enable Allow List and Enable Block List. Choose which lists you would like to use, then click the
+
below each list to add slots to it. Once you have added slots, enter the names of the semantic channels you would like to allow/exclude, one per line. In the following example, theground
channel is in an allowlist, so meshing will only capture the ground.
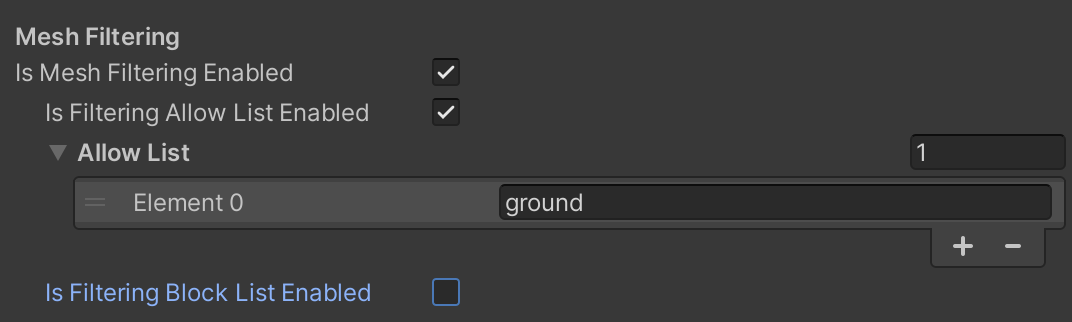
The allow/block lists will remember your settings and channel lists, even if you disable and re-enable them.
Recommended Usage
Semantic channels apply to a variety of common objects and structures, and there are some that you will usually not want included when creating a mesh. At minimum, we recommend excluding sky
and person
using a block list to keep those elements out of your mesh.
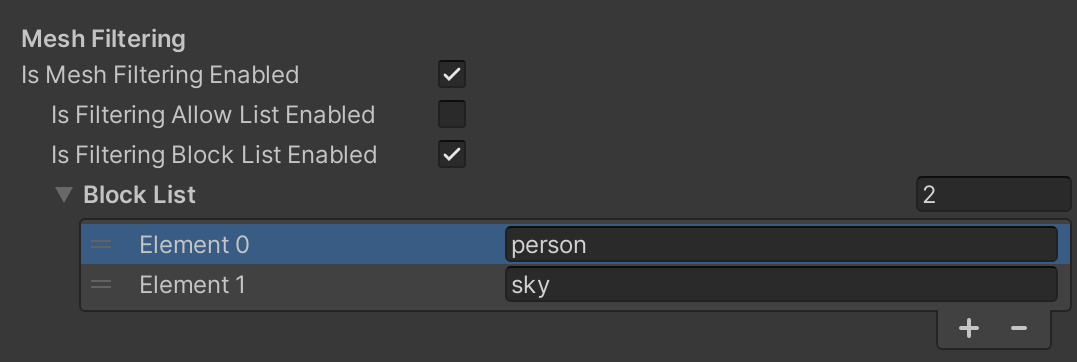
You can also combine allow lists and block lists to capture specific parts of a scene as a mesh. As an example, if you wanted to capture a mesh of a path through a grassy field, you could allow ground
while blocking sky
and grass
to only capture ground areas with no grass on them, resulting in a mesh of the path. For another example, if you wanted to capture a mesh of an interesting building, you could allow building
while blocking person
, ground
, and sky
to make sure the building is all that is captured.
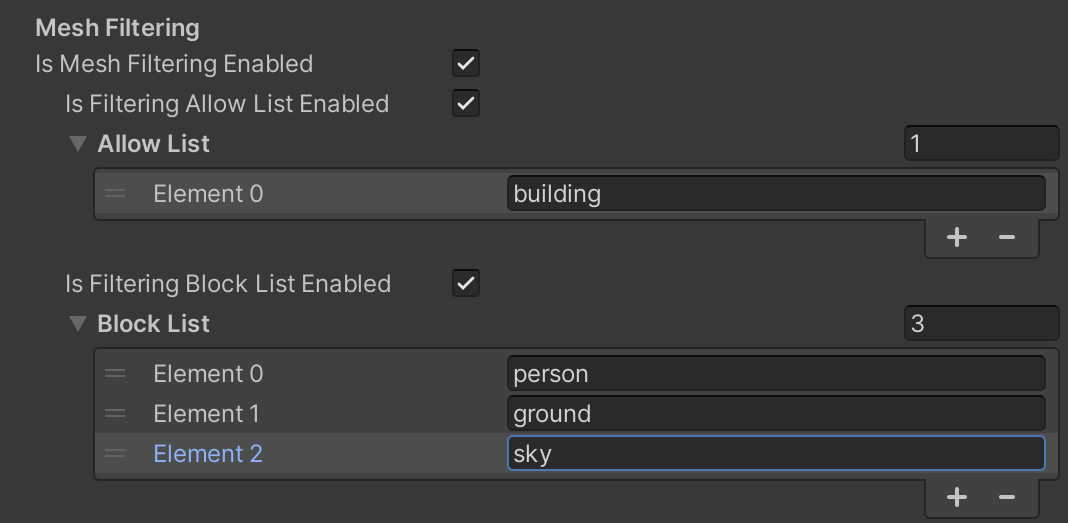
Script Example
This script demonstrates a basic example of how to use mesh filtering in code. It defines an allowlist and makes sure the list is active, then provides a method for turning mesh filtering on and off.
Click to reveal ToggleMeshFiltering.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using Niantic.Lightship.AR.Meshing
public class ToggleMeshFiltering : MonoBehaviour
{
[SerializeField] private LightshipMeshingExtension _meshingExtension;
// Start is called before the first frame update
void Start()
{
// Define the Allow List
_meshingExtension.AllowList = new List<string>() {"ground"};
_meshingExtension.IsFilteringAllowListEnabled = true;
}
void ToggleMeshFiltering()
{
_meshingExtension.IsMeshFilteringEnabled = !_meshingExtension.IsMeshFilteringEnabled;
}
}