How To Add Creature Navigation with Navigation Mesh
Navigation Mesh allows characters (known as Agents) to navigate the AR scene using point-and-click touch inputs.
Prerequisites
You will need a Unity project with Lightship AR and Lightship Meshing enabled. For more information, see Installing ARDK 3.0 and How to Create a Mesh and Add Physics.
You will also need a Unity GameObject
with the AR Mesh Manager and Lightship Meshing Extension Components. For more information, follow the Section 1 steps under Creating the Mesh.
Adding the Navigation Mesh Manager
To add a LightshipNavMeshManager
:
- Create an empty
GameObject
.- In the Hierarchy, select the root of the scene.
- From the main menu, select GameObject, then Create Empty Child.
- Name it NavMeshManager.
- Add a
LightshipNavMeshManager
component to the NavMeshManager object.- In the Inspector window, click Add Component.
- Type "LightshipNavMeshManager" in the search window, then select it.
- If you want to see the LightshipNavMesh, add a
LightshipNavMeshRenderer
component to NavMeshManager as well. This will render the LightshipNavMesh on-screen when the camera moves.- In the Inspector window, click Add Component again.
- This time, search for "LightshipNavMeshRenderer" instead, then select it.
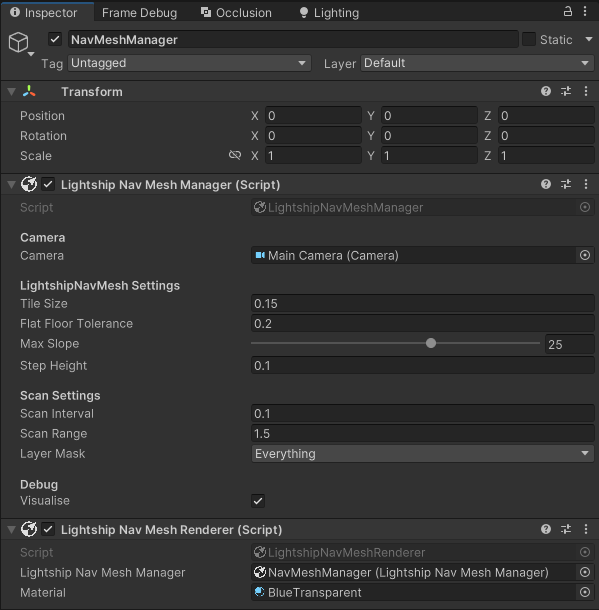
You can also add the LightshipNavMeshManager
prefab from the NavMesh sample project. For more information, see Sample Projects.
Adding an Agent to the LightshipNavMesh
To test out the LightshipNavMesh, we need to add an Agent to the scene to traverse it. The NavMesh sample project contains an Agent prefab that you can use for testing, but you may wish to create your own instead.
To create an Agent prefab:
- Add an object to the scene to act as an Agent.
- In the Hierarchy, select the root of the scene.
- Right-click, then select Create. For this example, choose Cube to add a basic cube as the testing Agent. Name it
TestAgent
.
- Add a
LightshipNavMeshAgent
component to the new Agent.- In the Hierarchy, select
TestAgent
. - In the Inspector window, click Add Component. Search for "LightshipNavMeshAgent", then select it to add the component.
- In the Hierarchy, select
- Add a
LightshipNavMeshAgentPathRenderer
component to show the path the Agent can take in the scene.- In the Hierarchy, select
TestAgent
. - In the Inspector window, click Add Component, then search for "LightshipNavMeshAgentPathRenderer" and select it to add the component.
- In the Hierarchy, select
- Drag the Agent to the Assets window to make a prefab, then remove it from the scene.
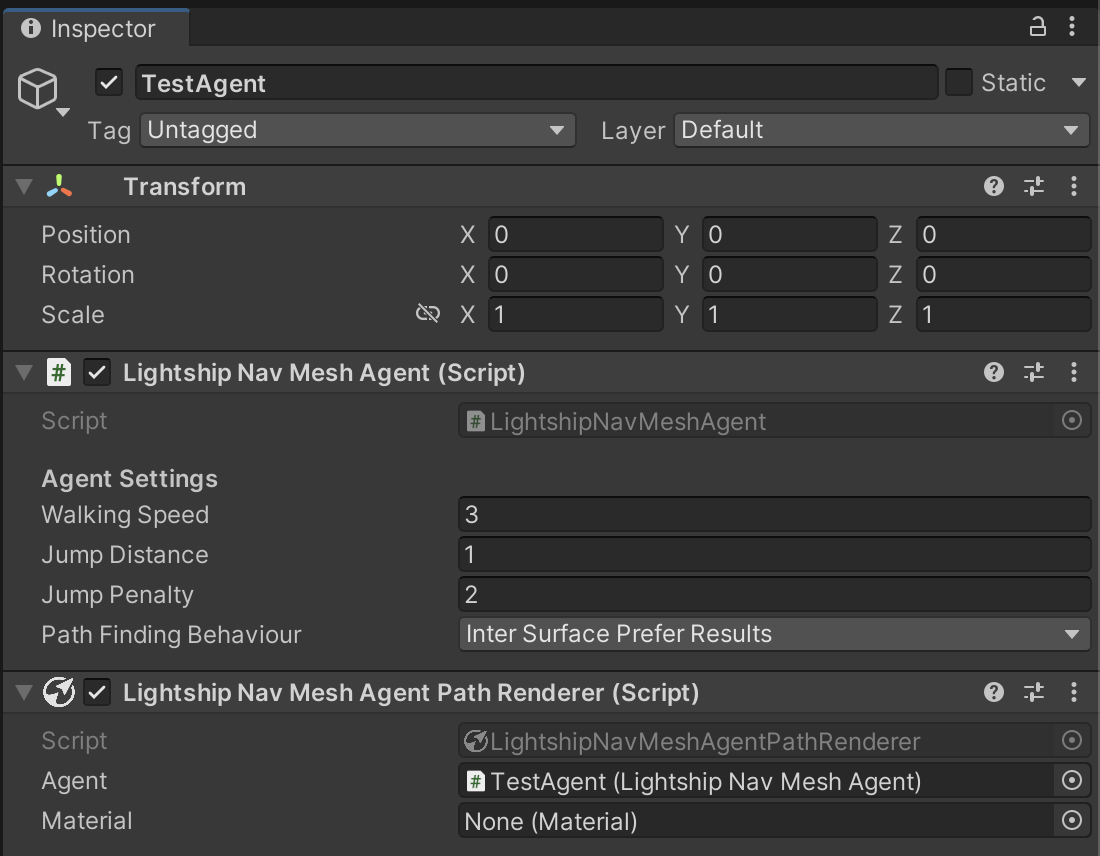
Create a Script to Control the Agent
To add a control script to the Agent:
- Add a script Asset.
- In the Assets window, right-click, then select Create, then C# Script.
- Name the new script
NavMeshHowTo
.
- Attach the script to the Agent.
- Select your Agent prefab, then click "Add Component" in the Inspector window.
- Search for "NavMeshHowTo", then select it to add the controller script to the prefab.
- Open
NavMeshHowTo.cs
, then copy in this sample script:
Click here to show the NavMeshHowTo script!
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using Niantic.Lightship.AR.NavigationMesh;
using UnityEngine.InputSystem;
/// SUMMARY:
/// LightshipNavMeshSample
/// This sample shows how to use LightshipNavMesh to add user driven point and click navigation.
/// When you first touch the screen, it will place your agent prefab.
/// Tapping a location moves the agent to that location.
/// The toggle button shows/hides the navigation mesh and path.
/// It assumes the _agentPrefab has LightshipNavMeshAgent on it.
/// If you have written your own agent type, either swap yours in or inherit from it.
///
public class NavMeshHowTo : MonoBehaviour
{
[SerializeField]
private Camera _camera;
[SerializeField]
private LightshipNavMeshManager _navmeshManager;
[SerializeField]
private GameObject _agentPrefab;
private GameObject _creature;
private LightshipNavMeshAgent _agent;
void Update()
{
HandleTouch();
}
public void ToggleVisualisation()
{
//turn off the rendering for the navmesh
_navmeshManager.GetComponent<LightshipNavMeshRenderer>().enabled =
!_navmeshManager.GetComponent<LightshipNavMeshRenderer>().enabled;
//turn off the path rendering on any agent
_agent.GetComponent<LightshipNavMeshAgentPathRenderer>().enabled =
!_agent.GetComponent<LightshipNavMeshAgentPathRenderer>().enabled;
}
private void HandleTouch()
{
//in the editor we want to use mouse clicks, on phones we want touches.
#if UNITY_EDITOR
if (Input.GetMouseButtonDown(0) || Input.GetMouseButtonDown(1) || Input.GetMouseButtonDown(2))
#else
//if there is a touch, call our function
if (Input.touchCount <= 0)
return;
var touch = Input.GetTouch(0);
//if there is no touch or touch selects UI element
if (Input.touchCount <= 0 )
return;
if (touch.phase == UnityEngine.TouchPhase.Began)
#endif
{
#if UNITY_EDITOR
Ray ray = _camera.ScreenPointToRay(Input.mousePosition);
#else
Ray ray = _camera.ScreenPointToRay(touch.position);
#endif
//project the touch point from screen space into 3d and pass that to your agent as a destination
RaycastHit hit;
if (Physics.Raycast(ray, out hit))
{
if (_creature == null )
{
_creature = Instantiate(_agentPrefab);
_creature.transform.position = hit.point;
_agent = _creature.GetComponent<LightshipNavMeshAgent>();
}
else
{
_agent.SetDestination(hit.point);
}
}
}
}
}